Nearly all of us have heard of or even used cURL. cURL is a powerful and incredibly useful command-line utility for tranferring data over network using various protocols.
One of the most common protocols to interact with is the HTTP/HTTPS protocol. This involves us making requests such as GET, POST, DELETE, PUT, etc.
The HTTP POST request is a type of request method that allows us to send data to a server. A POST request is responsible for a wide variety of actions such as submitting forms, payment authorization, and more.
You can learn more about the POST request below:
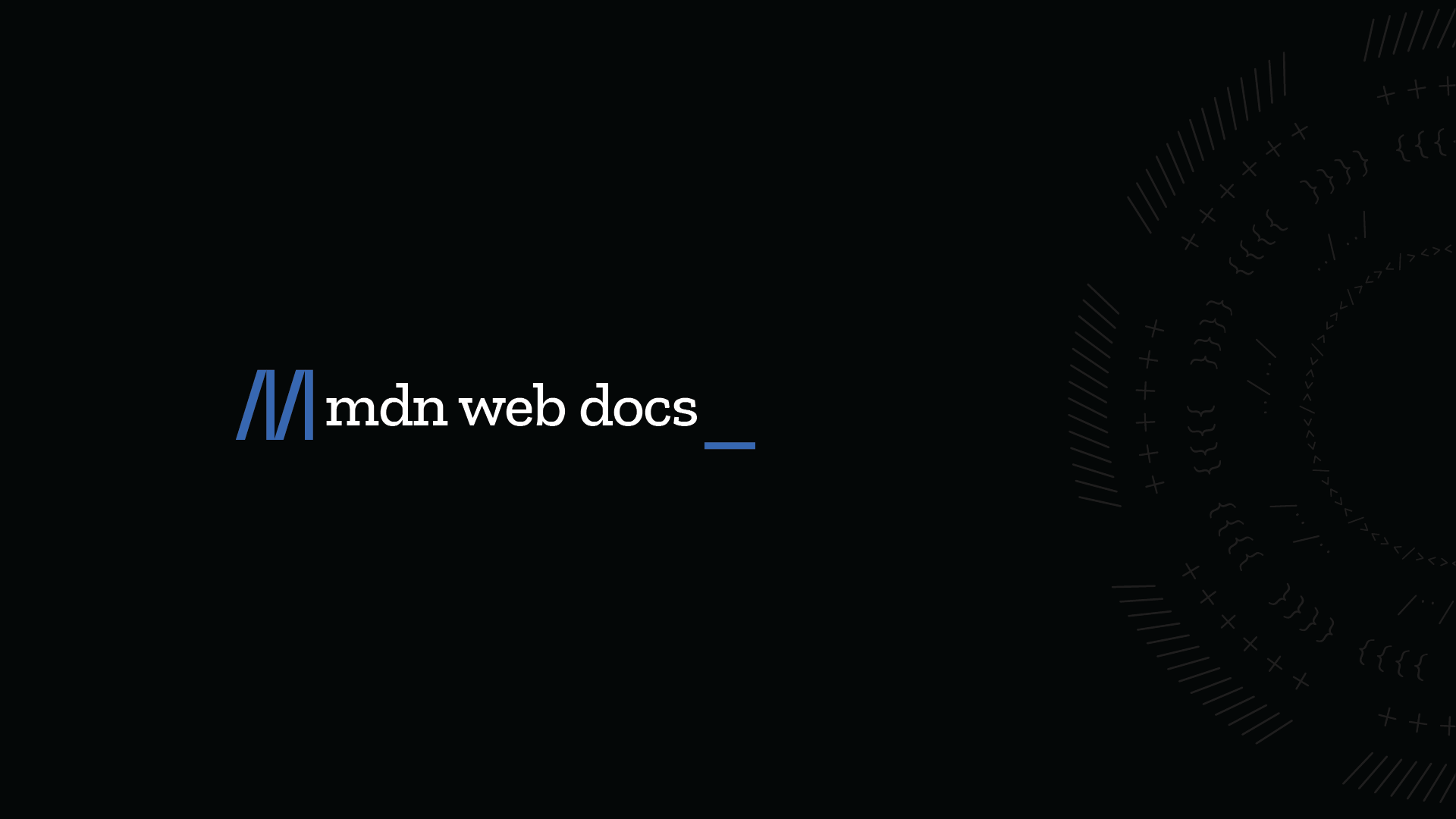
This is a fundamental part if you want to interact with APIs or automating some network tasks using cURL.
Command Syntax
The following shows the command syntax of sending a POST request using cURL.
curl -X POST [options] [URL]
In this case, the -X
option allows us to specify the type of request we wish to perform. In this case, we want to perform a HTTP POST
request.
An exampe POST request that contains data is as shown:
curl -X POST https://reqbin.com/echo/post/json
-H "Content-Type: application/json"
-d '{"server: "1", "auth": "true"}'
Pay attention to the command above. We start with the cURL command followed by the following options:
-X
- this option tells cURL that we want to perform an HTTP POST request.-d
- this parameter allows us to provide the associated data in the body of the HTTP request.-H
- this specifies the headers for the request. In our case, we specify the content type that we wish to send which is json data, expressed asapplication/json
.
You can use the the long-form versions of the above parameters. For example, you can replace the -X
with --request
and -H
with --headers
.
Examples
Let us explore various examples on how to perform various types of POST requests when working in cURL.
Example 1 - Post Request Body
The first exampe is post data on the body of a request message using cURL. As you saw in the first example, we specify the data using the -d
option as shown:
curl -X POST https://example.com/api/v2/hi
-H "Content-Type: application/json"
-d '{"name": "csalem", "url": "geekbits.io"}'
NOTE: To post JSON data using cURL, we need to set the Content-Type
to application/json
and use the -d
parameter to pass JSON to cURL
Example 2 - Post File.
cURL does allow us to post a file using the combination of the -d
and the -F
parameters. We then need to start the data using the @
symbol.
By default, cURL will infer the content type from the file extension.
curl -d @schema.json https://example.com/api/v2/db
This will use the data from the file specified in the command above.
Example 3 - Post XML with cURL.
We can also post XML data to the server using the -d
option and specifying the content type as application/xml
.
An example is as shown:
curl -X POST https://example.com/api/v2/xml
-H "Content-Type: application/xml"
-d "<Data><UserID>1</UserID><Username>geekbits</Username></Data>"
Example 4 - Sending Auth Creds
If you are dealign with basic authentication endpoint, you can pass the username and password credentials using the --user
option as shown:
curl -X POST https://example.com/api/v2/dc
--user "admin:password"
cURL will automatically encrypt the specified user credentails into base63 encoded string before passing the into the server by using the Authorization: Basic [token]
header.
Other POST Options
The following are other options and parameters you can use when sending POST requests using cURL.
-X --requests <method> Specify request method
-b --cookie <data|filename> Specify where to write cookies
-c --cookie-jar <filename> Specify cookie storage file
-d --data <data> Send data in POST request
-f --fail Fail fast on server errors
-F --form <name=content> Form data for POST
-H --header <header/@file> Include header in request
-i --include Include HTTP headers
-l --head Fetch headers only
-k --insecure Skip secure connection verification
-L --location Force redirect follow
-o --output <file> Write output to file
-O --remote-name Write output to local file named like remote file
-s --silent Do not show progress or errors
-v --verbose Make output verbose
-w --write-out <format> Display information on stdout after transfer
:)
Conclusion
In this tutorial, we learned the fundamentals of working with and sending POST requests using cURL and the various options we can use to customize the behavior of cURL.